In the development of modern web applications, rendering plays a pivotal role in determining how content is delivered to users. Two primary rendering strategies, Server-Side and Client-Side Rendering, offer distinct benefits and trade-offs. Whether you’re building a static website, a dynamic e-commerce platform, or an interactive single-page application (SPA), understanding the differences between these rendering techniques is crucial for optimizing user experience, search engine optimization (SEO), and performance.
In this article, we explore the concepts of SSR and CSR, examining how they work, their benefits, and the best use cases for each approach.
What is Server-Side Rendering (SSR)?
Server-Side Rendering (SSR) refers to the process where the server generates the complete HTML content for a webpage before sending it to the client’s browser. In this model, React (or another JavaScript framework) renders components on the server, and the fully rendered HTML page is delivered to the client, ready for immediate display.
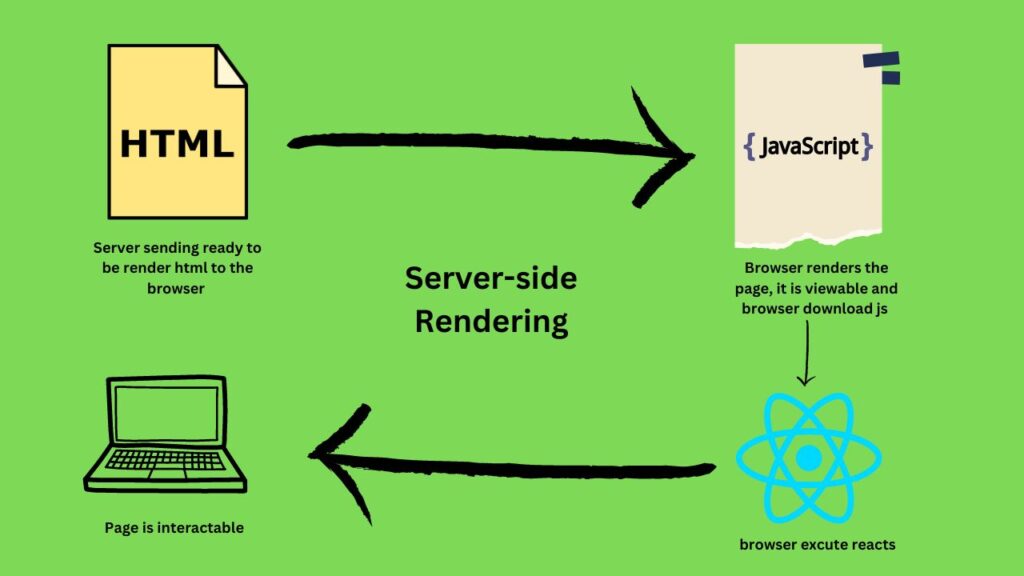
How Does SSR Work?
- Initial Request: The client (browser) sends a request for the webpage to the server.
- Server Processing: The server processes this request, renders the React components, and generates the complete HTML markup.
- HTML Sent to Client: The server sends the rendered HTML to the client’s browser.
- Immediate Display: The browser displays the content immediately upon receiving the HTML.
- JavaScript Hydration: After the HTML content is displayed, JavaScript loads and attaches event listeners and interactivity to the page through a process called “hydration.”
This method provides several benefits, particularly in situations where speed, SEO, and user experience are of paramount importance.
Benefits of Server-Side Rendering (SSR)
1. Faster Initial Page Load
Because the server sends fully rendered HTML, users can see content as soon as the page is loaded, without waiting for JavaScript execution. This can be especially beneficial for users on slow internet connections or using low-powered devices. The initial load time is fast because the browser does not need to process a lot of JavaScript before showing the content.
2. SEO Optimization
Search engines like Google rely on easily accessible HTML content to index web pages correctly. With SSR, the full HTML is available to search engines right from the start, improving the chances of better search rankings. Pages rendered on the server are also easier for bots to crawl, which is essential for content-driven websites that rely on SEO for visibility.
3. Improved Performance for Low-Powered Devices
Since most of the rendering is done on the server, less processing is required on the client-side. This can improve the performance of the website on older or less powerful devices, as the heavy lifting of rendering is done on the server.
Use Cases for SSR
- Content-Driven Websites: Websites that are heavily focused on displaying content, such as blogs, news platforms, and media websites, benefit greatly from SSR. SEO and fast initial page load times are essential for these types of websites.
- E-commerce Platforms: In e-commerce, speed and user experience are crucial for customer retention. Faster page loads reduce bounce rates, and better SEO ensures higher visibility.
- Any Website Requiring SEO: Websites that depend on search engines for traffic should opt for SSR to ensure they are crawlable and rank well in search results.
The Restaurant Analogy for SSR
Imagine being at a restaurant where the chef prepares your entire meal in the kitchen and serves it ready-to-eat at your table. You don’t need to wait for any additional steps or do any work; everything is ready for you to consume immediately. This is how SSR works: the server (chef) does all the heavy lifting, and the client (your browser) gets a fully cooked webpage (meal) ready to be displayed instantly.
What is Client-Side Rendering (CSR)?
Client-Side Rendering (CSR) shifts the burden of rendering from the server to the client’s browser. With CSR, the server sends minimal HTML content to the browser, and JavaScript takes over the task of rendering the entire web page on the client-side. React, for instance, renders components dynamically in the browser after the initial load, and any future updates happen directly in the browser without requiring a full page reload.
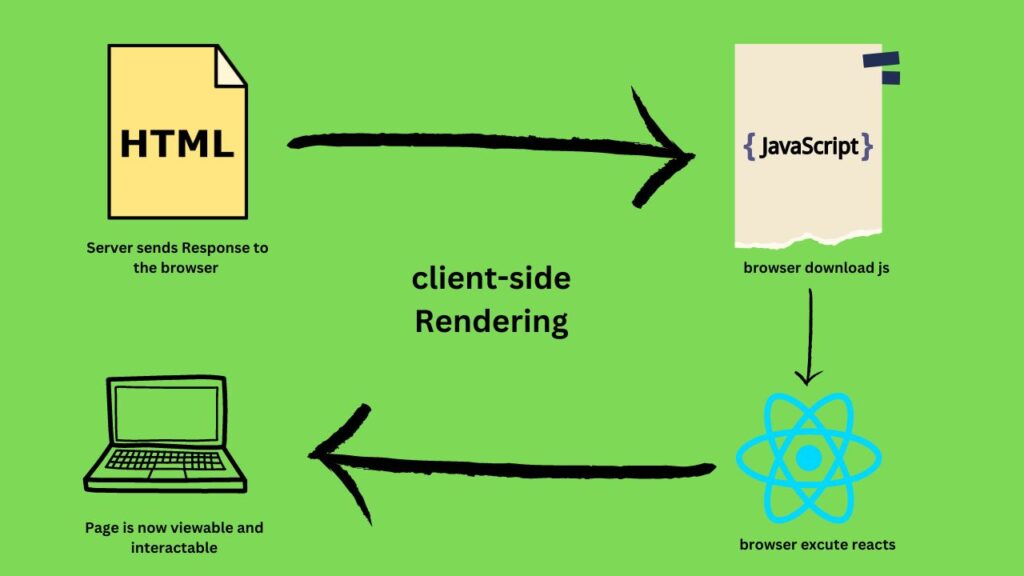
How Does CSR Work?
- Initial Request: The client (browser) sends a request for the webpage to the server.
- Server Response: The server sends a minimal HTML file, often containing a simple
div
element and a link to the JavaScript bundle. - JavaScript Execution: The browser downloads the JavaScript file, which then renders the page’s content dynamically on the client-side.
- Page Display: Once the JavaScript is executed, the content appears in the browser.
CSR is commonly used in single-page applications (SPAs), where the goal is to provide a fast, interactive experience after the initial page load.
Benefits of Client-Side Rendering (CSR)
1. Faster Subsequent Interactions
After the initial page load, client-side applications can offer faster and more seamless interactions. Instead of requesting new pages from the server, CSR applications only fetch data, making the user experience more fluid and dynamic.
2. Enhanced Interactivity
CSR allows for highly interactive web applications, where content updates dynamically in response to user interactions. This is particularly useful for dashboards, data-driven applications, and social media platforms, where real-time updates are critical.
3. Reduced Server Load
Because most of the rendering happens in the client’s browser, CSR reduces the processing load on the server. For applications with a large number of users, this can lead to better scalability and performance from the server’s perspective.
Use Cases for CSR
- Single-Page Applications (SPAs): Applications that require constant interaction and data updates, like social media platforms, messaging apps, or project management tools, benefit from CSR. The user experience becomes smoother and more interactive after the initial page load.
- Data-Driven Applications: Dashboards and analytics platforms that constantly display and refresh data are ideal candidates for CSR, as users need real-time updates without waiting for server refreshes.
- Real-Time Applications: CSR is perfect for applications that rely on real-time updates and interactions, such as collaborative tools, stock trading platforms, and messaging apps.
The Restaurant Analogy for CSR
Imagine going to a different restaurant where the chef (server) only brings a plate of raw ingredients (minimal HTML). You, the customer (your browser), are given a small kitchenette (JavaScript) to cook the meal yourself. While this takes more time initially, once you’re done, you have full control over how the meal is prepared and can make changes whenever you want without having to wait for the chef again.
SSR vs. CSR: Key Differences
Aspect | SSR (Server-Side Rendering) | CSR (Client-Side Rendering) |
---|---|---|
Initial Load Time | Faster (fully rendered HTML sent to the client) | Slower (minimal HTML, JavaScript runs to load content) |
SEO | Excellent for SEO; fully rendered HTML is available for search engine crawlers | Limited SEO as content is rendered dynamically via JavaScript |
Interactivity | May require page reloads for interactivity | Highly interactive and dynamic after the initial load |
Server Load | Higher server load due to rendering on the server | Reduced server load as rendering occurs on the client-side |
Use Cases | Content-driven websites, e-commerce, SEO-heavy sites | Interactive SPAs, dashboards, real-time applications |
Read About: The 9 Most Important Types of Algorithms to Know for Coding Interviews.
Choosing Between SSR and CSR
Choosing between SSR and CSR depends on your project’s needs. If fast initial loads and SEO are priorities, SSR may be the right approach. For highly interactive, data-driven applications, CSR can offer a more dynamic user experience.
Many modern applications combine both techniques to get the best of both worlds. Hybrid approaches such as hydration allow developers to render the initial HTML on the server (SSR) and then hand over dynamic interactions to the client (CSR), creating a seamless user experience.
Conclusion: Server-Side and Client-Side Rendering
Both Server-Side Rendering and Client-Side Rendering have their strengths and weaknesses. While SSR shines in areas like fast initial loading and SEO, CSR excels in interactivity and reducing server load. The right choice depends on the needs of your application, the user experience you want to create, and the resources available for development. For some, a hybrid approach might be the best way forward, combining the power of both SSR and CSR.